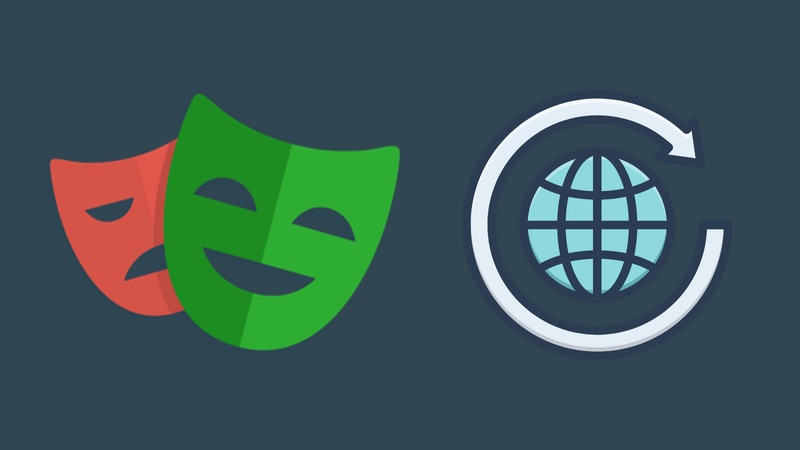
To refresh a page in Playwright, we can use the page.reload() method, which will reload the current page. This method reloads the current page in the same way as if the user had triggered a browser refresh.
Table of Contents
Syntax
await page.reload();
await page.reload(options);
Arguments
- options Object (optional)
- timeout number (optional): Maximum operation time in milliseconds. Defaults to 0 - no timeout. The default value can be changed via:
- navigationTimeout option in the config,
- or by using the following methods:
- browserContext.setDefaultNavigationTimeout(),
- browserContext.setDefaultTimeout()
- page.setDefaultNavigationTimeout()
- or page.setDefaultTimeout() methods.
- waitUntil "load"|"domcontentloaded"|"networkidle"|"commit" (optional)#: When to consider operation succeeded, defaults to load. Events can be either:
- 'domcontentloaded' - consider operation to be finished when the DOMContentLoaded event is fired.
- 'load' - consider operation to be finished when the load event is fired.
- 'networkidle' - DISCOURAGED consider operation to be finished when there are no network connections for at least 500 ms. Don't use this method for testing, rely on web assertions to assess readiness instead.
- 'commit' - consider operation to be finished when network response is received and the document started loading.
- timeout number (optional): Maximum operation time in milliseconds. Defaults to 0 - no timeout. The default value can be changed via:
Returns
- Promise<null|Response>#
The page.reload() method returns the main resource response. In case of multiple redirects for a URL, the navigation will resolve with the response of the last redirect.
Example
For the demo, let's fill input-1 with the value Hello and input-2 with the World.
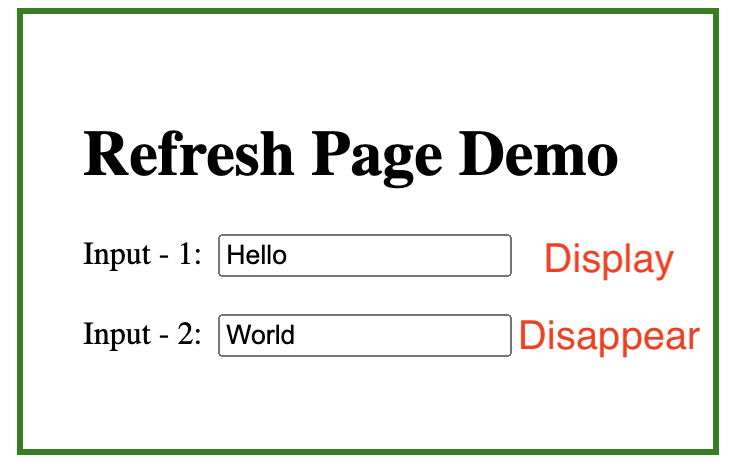
Once you refresh the page, Hello will be displayed in input-1, and the value World will disappear in input-2.
Code
test.only('Refresh Browser Page', async ({ page }) => {
await page.goto('http://autopract.com/selenium/refresh/');
// Fill in Input Value
await page.locator("#display").type('Hello');
await page.locator("#clear").type('World') ;
// Reload or Refresh Page
await page.reload();
// Get value of both inputs
const input1 = await page.locator("#display").inputValue();
const input2 = await page.locator("#clear").inputValue();
// Verify Values After Refresh
expect(input1).toEqual("Hello");
expect(input2).toEqual("");
})