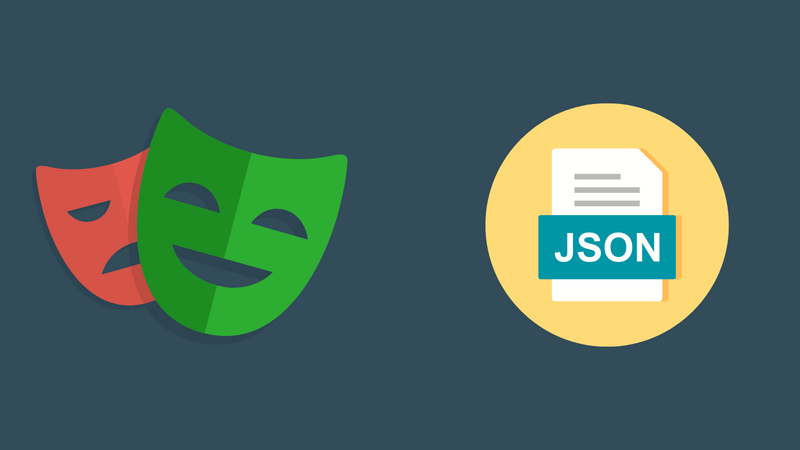
In this article, let's discuss how to read test data from JSON files of different formats.
Table of Contents
Read Single Object
Let's start with a simple example.
{
"username":"tarun",
"password":"123456"
}
Here, we have a single object with two properties: username and password. Each property has a corresponding value: the username key value is tarun, and the password value is 123456.
Here, to read data, we require two steps.
Step 1: Import File
TypeScript
import myuserdata from '../../testdata/userdata.json';
JavaScript
const myuserdata = require('../../testdata/userdata.json');
Step 2: Access properties using the import variable and key.
myuserdata.username
myuserdata.password
Code
import myuserdata from '../../testdata/userdata.json';
import { test } from '@playwright/test';
test('Read Single JSON Record', async ({ page }) => {
console.log(myuserdata.username);
console.log(myuserdata.password);
})
Read Array of Objects
In the previous example, we had a single record; now, let's see how to read multiple records of the same type.
[
{
"username":"tarun",
"password":"12345"
},
{
"username":"ram",
"password":"67890"
}
]
Code
import myuserdata from '../../testdata/userdata-array.json';
import { test, expect } from '@playwright/test';
test('Read JSON Array', async ({ page }) => {
console.log(myuserdata[1].username);
console.log(myuserdata[1].password);
})
Here import statement is same, but we have used array index 1 to read the 2nd record with username ram and password 67890.
Read Array of Objects with Key
In our previous example, the array does not have a name or key, but you will most likely find the nested JSON where the array name or key is given.
{
"users":[
{
"username":"tarun@example.com",
"password":"12345"
},
{
"username":"ram@example.com",
"password":"67890"
}
]
}
Code
import myuserdata from '../../testdata/userdata-array-name.json';
import { test, expect } from '@playwright/test';
test('Read JSON Array Name', async ({ page }) => {
console.log(myuserdata.users[1].username);
console.log(myuserdata.users[1].password);
})
Now, myuserdata will represent the whole JSON; users will represent the array, 1 is index for the 2nd record in users array, and username and password are properties of the 2nd object in the array. We can also read it like this:
import { users } from '../../testdata/userdata-array-name.json';
import { test } from '@playwright/test';
test('Read JSON Array With Name 2', async ({ page }) => {
console.log(users[1].username);
console.log(users[1].password);
})
Observe the change in the import statement; myuserdata is replaced by {users}. Also while logging in console, myuserdata is removed, Here, we are directly referring to an array using its name.